Making downloadable QR Code Generator With Flutter
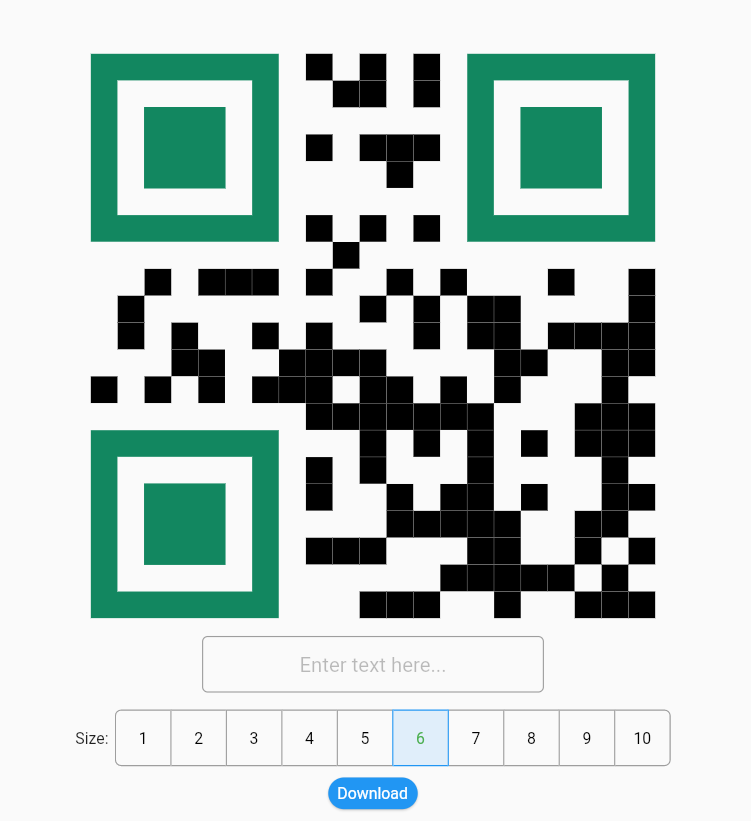
One of my side web projects, I needed to use QR Code generator. There are many samples on web but I wanted it to be downloadable. With Flutter I should find the widget and should be saved as Image. At this point RepaintBoundary comes to help. With this widget I captured the widget as Image and to make it downloadable, I used dart:html.
To capture widget as Image
Future<void> _capturePng() async {
final picData = await _painter.toImageData((size * 100).toDouble(),
format: ImageByteFormat.png);
await writeToFile(picData);
}
and to make it downloadable;
Future<void> writeToFile(ByteData data) async {
final bytes =
data.buffer.asUint8List(data.offsetInBytes, data.lengthInBytes);
final blob = html.Blob([bytes]);
final url = html.Url.createObjectUrlFromBlob(blob);
final anchor = html.document.createElement('a') as html.AnchorElement
..href = url
..style.display = 'none'
..download = 'qr_code.png';
html.document.body.children.add(anchor);
anchor.click();
html.document.body.children.remove(anchor);
html.Url.revokeObjectUrl(url);
}
You can see full code from github link;
Read other posts