Error handling by using middlewares in .NET5 Web API
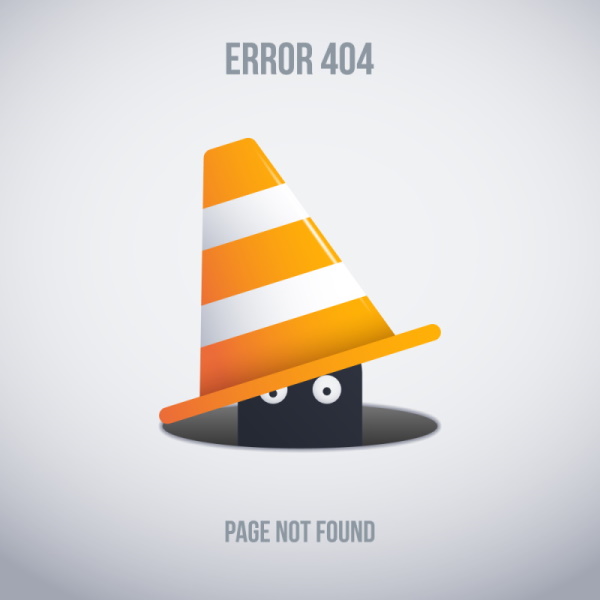
Actually this error handling implementation is not specific for .NET5, it can be used on core versions as well but title became more attractive 😜 I needed an error handling implementation on some web apis, and middlewares became best solution for me. Let me tell what I did.
I created a new middleware for error handling. This implementation works as global error handling. I can both log the error and send the error as a response to the client. Power of middlewares make me excited.
MiddleWare class
public class ErrorLoggingMiddleware
{
private readonly RequestDelegate _next;
private readonly ILogger<ErrorLoggingMiddleware> _logger;
public ErrorLoggingMiddleware(RequestDelegate next, ILogger<ErrorLoggingMiddleware> logger)
{
_next = next;
_logger = logger;
}
public async Task Invoke(HttpContext context)
{
try
{
await _next(context);
}
catch (Exception ex)
{
_logger.LogError(ex, "Something wrong while invoking request {request} with API {requestAPI}", context.Request?.Method, context.Request?.Path);
await SendErrorResponse(context, ex);
}
}
private Task SendErrorResponse(HttpContext context, Exception ex)
{
context.Response.StatusCode = StatusCodes.Status500InternalServerError;
context.Response.ContentType = "application/json";
return context.Response.WriteAsync(new Response(new Error {Message = ex.Message }).ToString());
}
}
And let’s register our error handling middleware to the application builder by using extension methods. I created a static class for middlewares;
public static class MiddlewareExtensions
{
public static IApplicationBuilder UseErrorLogging(this IApplicationBuilder builder)
{
return builder.UseMiddleware<ErrorLoggingMiddleware>();
}
}
Next will be about caching middlewares by using attributes.
See you next middleware post 😄
Read other posts